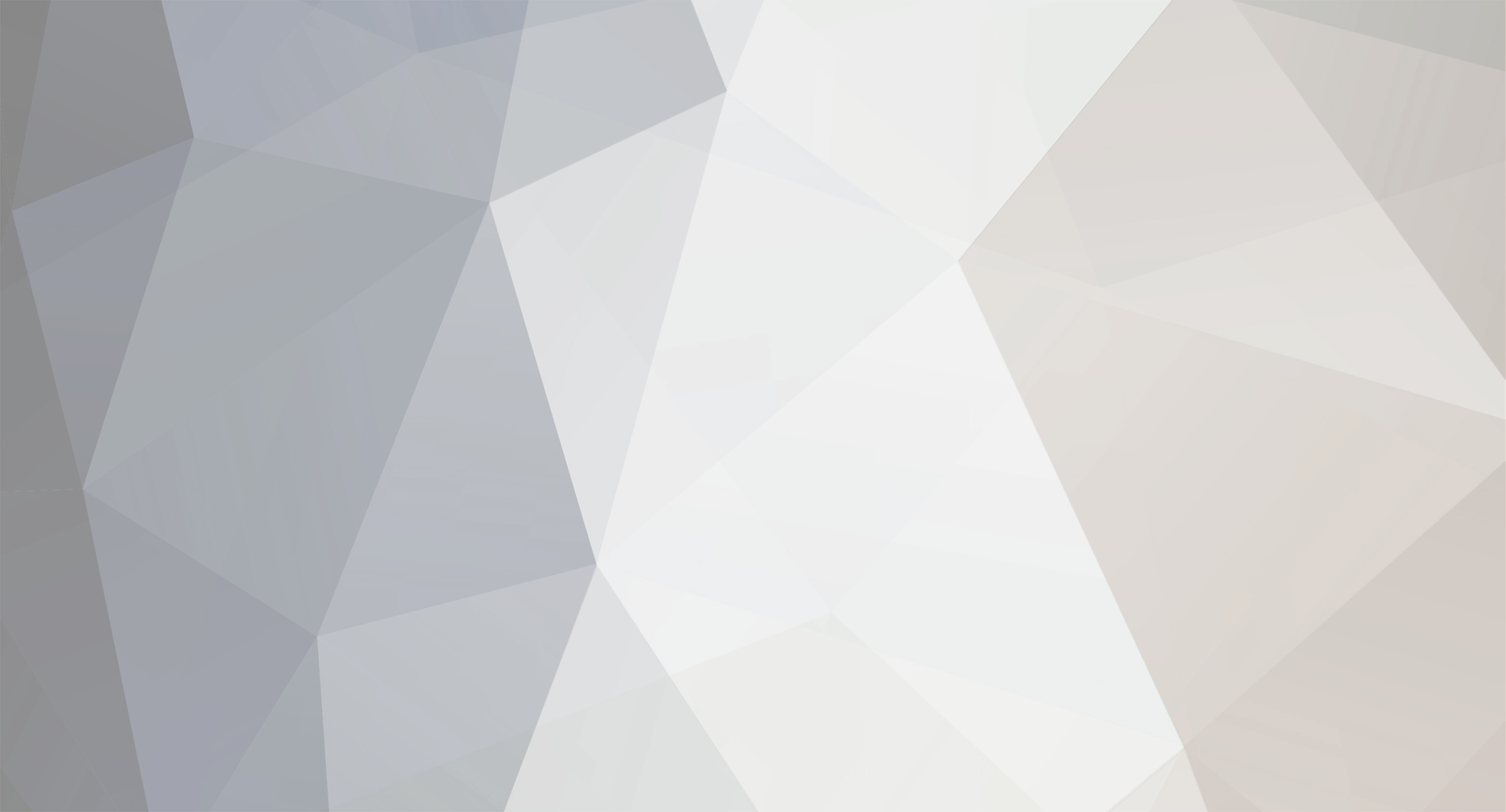
baltostar
Member-
Posts
37 -
Joined
-
Last visited
Content Type
Profiles
News & Announcements
Zombies Library
Easter Egg Guides
Intel
Forums
Everything posted by baltostar
-
I disagree with part of your statement. When talking about the code, all we see are the scripts. There are a lot of things hidden in the maps that are not in the scripts. I do agree looking at the code is cheating, however I don't see any harm in looking at it to verify that what has been found is the completed form of the Easter egg. (Though I wish the would encrypt the scripts as well, that way people don't cheat and post all the steps when the game is first released.)
-
I don't recommend this for a starting language. Its something that COD uses for their games. If you want to learn coding, I recommend you taking a java class. The code for this game is written more in a C Language style as they use Pointers to function mapping. You might want to google, Function, Method, and Struct.
-
I personally don't see anything in code that would support the idea of shooting the soul and here is my justification: thundergun_check( model, trig, weapon_combo_spot ) { model endon( "death" ); while (1) { self waittill( "weapon_fired" ); if ( self GetCurrentWeapon() == "thundergun_upgraded_zm" ) { if ( DistanceSquared( self.origin, weapon_combo_spot.origin ) vector_to_spot = VectorNormalize( weapon_combo_spot.origin - self GetWeaponMuzzlePoint() ); vector_player_facing = self GetWeaponForwardDir(); angle_diff = acos( VectorDot( vector_to_spot, vector_player_facing ) ); if ( angle_diff flag_set( "thundergun_hit" ); RadiusDamage( trig.origin, 5, 1, 1, self ); } } } } } In this section of code you can see the math on aiming the thunder gun toward the gursh. No where else in the code is similar logic used. For your convenience, I have posted the soul release code below to show how it moves. soul_release( model, origin ) { soul = Spawn( "script_model", origin ); soul SetModel( "tag_origin" ); soul PlayLoopSound( "zmb_egg_soul" ); fx = PlayFXOnTag( level._effect["gersh_spark"], soul, "tag_origin" ); time = 20; model waittill( "death" ); level thread play_egg_vox( "vox_ann_egg6_success", "vox_gersh_egg6_success", 9 ); level thread wait_for_gersh_vox(); soul MoveZ( 2500, time, time - 1 ); wait( time ); soul Delete(); wait(2); level thread samantha_is_angry(); }
-
activate_casimir_light( num ){ spot = GetStruct( "casimir_light_"+num, "targetname" ); if ( IsDefined( spot ) ) { light = Spawn( "script_model", spot.origin ); light SetModel( "tag_origin" ); light.angles = spot.angles; fx = PlayFXOnTag( level._effect["fx_zmb_light_floodlight_bright"], light, "tag_origin" ); level.casimir_lights[ level.casimir_lights.size ] = light; } } As seen in this code, the casimir light is a zombie flood light. spot is the location of the casimir light being looked up by the name of the location defined. It spawns a model that is a light at the location just looked up. The if (IsDefined( spot)) is not needed, but would allow the struct of the casimir light to be programmed in later and added to the code without having to update this script. "fx =" does nothing "PlayFXOnTag( level._effect["fx_zmb_light_floodlight_bright"], light, "tag_origin" );" gets the flood light effect and applies it to the now configured model "light", playing the effect at the origin of the model "light". "level.casimir_lights[ level.casimir_lights.size ] = light;" sets the model into an array so it can be latter cleaned up in the following function: weapon_combo_event() { flag_init( "thundergun_hit" ); weapon_combo_spot = GetStruct( "weapon_combo_spot", "targetname" ); focal_point = Spawn( "script_model", weapon_combo_spot.origin ); focal_point SetModel( "tag_origin" ); focal_point PlayLoopSound( "zmb_egg_notifier", 1 ); fx = PlayFXOnTag( level._effect["gersh_spark"], focal_point, "tag_origin" ); level.black_hold_bomb_target_trig = Spawn( "trigger_radius", weapon_combo_spot.origin, 0, 50, 72 ); level.black_hole_bomb_loc_check_func = ::bhb_combo_loc_check; flag_wait( "weapons_combined" ); level.black_hold_bomb_target_trig Delete(); level.black_hole_bomb_loc_check_func = undefined; focal_point Delete(); for ( i=0; i { level.casimir_lights[i] Delete(); } }
-
Found a design artifact that shows that in solo mode zombies, the user would only loose one perk, but I don't know the reason why this code was written or when it would be called. There is no reference to this function that I can find in any of the back ops code I have. Source: _zombiemode_deathcard.gsc //----------------------------------------------------------------------------------- // solo player gets both weapons back and only loses 1 perk //----------------------------------------------------------------------------------- deathcard_give_solo() { dc = level.deathcards[0]; // perks self maps\_zombiemode::laststand_giveback_player_perks(); take = true; startWeapon = dc.weapon[dc.current_weapon]; if ( startWeapon == "m1911_zm" ) { take = false; } currentWeapon = self GetCurrentWeapon(); if ( currentWeapon != "none" && take ) { self TakeWeapon( currentWeapon ); } for ( i = 0; i { if ( IsDefined( dc.weapon[i] ) ) { weapon = dc.weapon[i]; if ( weapon != "m1911_zm" || take ) { self GiveWeapon( weapon, 0 ); self SetWeaponAmmoClip( weapon, WeaponClipSize( weapon ) ); self GiveStartAmmo( weapon ); } } } if ( dc.current_weapon >= 0 && take ) { self SwitchToWeapon( dc.weapon[dc.current_weapon], 0 ); } // score //self.old_score += dc.score; //self.score += dc.score; // abilities //if ( IsDefined( dc.curr_ability ) ) //{ // self maps\_zombiemode_ability::giveAbility( dc.curr_ability ); // self maps\_zombiemode_ability::update_player_ability_status(); //} if ( maps\_zombiemode_weap_cymbal_monkey::cymbal_monkey_exists() ) { if ( dc.zombie_cymbal_monkey_count ) { self giveweapon( "zombie_cymbal_monkey" ); self setweaponammoclip( "zombie_cymbal_monkey", dc.zombie_cymbal_monkey_count ); } } }
-
looking at the _zombie mode.gsc file, I found this line to exclude all perks from displaying low ammo. Just more of an interesting find but doesn't provide much incite. if(!isDefined(weap) || weap == "none" || weap == "zombie_perk_bottle_doubletap" || weap == "zombie_perk_bottle_jugg" || weap == "zombie_perk_bottle_revive" || weap == "zombie_perk_bottle_sleight" || weap == "mine_bouncing_betty" || weap == "claymore_zm" || weap == "syrette_sp" || weap == "zombie_knuckle_crack" || weap == "zombie_bowie_flourish" || issubstr( weap, "knife_ballistic_" ) || ( GetSubStr( weap, 0, 3) == "gl_" ) ) Source: track_players_ammo_count() { self endon("disconnect"); self endon("death"); wait(5); while(1) { players = get_players(); for(i=0;i { if(!IsDefined (players[i].player_ammo_low)) { players[i].player_ammo_low = 0; } if(!IsDefined(players[i].player_ammo_out)) { players[i].player_ammo_out = 0; } weap = players[i] getcurrentweapon(); //iprintln("current weapon: " + weap); //iprintlnbold(weap); //Excludes all Perk based 'weapons' so that you don't get low ammo spam. if(!isDefined(weap) || weap == "none" || weap == "zombie_perk_bottle_doubletap" || weap == "zombie_perk_bottle_jugg" || weap == "zombie_perk_bottle_revive" || weap == "zombie_perk_bottle_sleight" || weap == "mine_bouncing_betty" || weap == "claymore_zm" || weap == "syrette_sp" || weap == "zombie_knuckle_crack" || weap == "zombie_bowie_flourish" || issubstr( weap, "knife_ballistic_" ) || ( GetSubStr( weap, 0, 3) == "gl_" ) ) { continue; } //iprintln("checking ammo for " + weap); if ( players[i] GetAmmoCount( weap ) > 5) { continue; } if ( players[i] maps\_laststand::player_is_in_laststand() ) { continue; } else if (players[i] GetAmmoCount( weap ) 0) { if (players[i].player_ammo_low != 1 ) { players[i].player_ammo_low = 1; players[i] maps\_zombiemode_audio::create_and_play_dialog( "general", "ammo_low" ); players[i] thread ammo_dialog_timer(); } } else if (players[i] GetAmmoCount( weap ) == 0) { if(!isDefined(weap) || weap == "none") { continue; } wait(2); if( players[i].player_ammo_out != 1 ) { players[i].player_ammo_out = 1; players[i] maps\_zombiemode_audio::create_and_play_dialog( "general", "ammo_out" ); players[i] thread ammoout_dialog_timer(); } } else { continue; } } wait(.5); } }
-
HoboRisesAgain, if you look at the second code block that you quoted, it is rather clear that "level thread soul_release( self, trig.origin );" is only called when ray gun, thunder gun, and dolls have hit the gursh device. Cross bow is set true in the code, but is never used within the scope of the function.
-
This thread was created after the video was made. I can only analyze the code and give you my view on it. I never said you had to agree with me. People should use there own judgment on what they read. Note that I never took credit for finding this error in code. I my self over looked it. That is why I gave a complement to the poster of this thread.
-
Please the code in question as I can't find any reference to zombie_flood_light_bright in the code. I am not sure what you don't understand. I'm posting the code outside of a code block so I can show you the ending sequence: soul_release( model, origin ) { soul = Spawn( "script_model", origin ); soul SetModel( "tag_origin" ); soul PlayLoopSound( "zmb_egg_soul" ); fx = PlayFXOnTag( level._effect["gersh_spark"], soul, "tag_origin" ); time = 20; model waittill( "death" ); level thread play_egg_vox( "vox_ann_egg6_success", "vox_gersh_egg6_success", 9 ); level thread wait_for_gersh_vox(); soul MoveZ( 2500, time, time - 1 ); wait( time ); soul Delete(); wait(2); level thread samantha_is_angry(); } wait_for_gersh_vox() { wait(12.5); players = GetPlayers(); for ( i=0; i { players thread reward_wait(); } } reward_wait() { while ( !is_player_valid( self ) || ( self UseButtonPressed() && self in_revive_trigger() ) ) { wait( 1.0 ); } level thread maps\_zombiemode_powerups::minigun_weapon_powerup( self, 90 ); }
-
I reviewed the question code and have determined why cross bow doesn't matter: wait_for_combo( trig ) { self endon( "death" ); self thread kill_trig_on_death( trig ); weapon_combo_spot = GetStruct( "weapon_combo_spot", "targetname" ); ray_gun_hit = false; doll_hit = false; crossbow_hit = false; players = get_players(); array_thread( players, ::thundergun_check, self, trig, weapon_combo_spot ); while ( 1 ) { trig waittill( "damage", amount, attacker, dir, org, mod ); if ( isDefined( attacker ) ) { if ( mod == "MOD_PROJECTILE_SPLASH" && (attacker GetCurrentWeapon() == "ray_gun_upgraded_zm" ) ) { ray_gun_hit = true; } else if ( mod == "MOD_GRENADE_SPLASH" ) { if ( amount >= 90000 ) { doll_hit = true; } else if ( attacker GetCurrentWeapon() == "crossbow_explosive_upgraded_zm" ){ crossbow_hit = true; } } if ( ray_gun_hit && doll_hit && flag( "thundergun_hit" ) ) { flag_set( "weapons_combined" ); level thread soul_release( self, trig.origin ); return; } } } } Unlike ray_gun_hit and doll_hit, crossbow_hit is never used. The logic was put in to set it true but as seen here, it is never referenced to end the Easter egg: if ( ray_gun_hit && doll_hit && flag( "thundergun_hit" ) ) { flag_set( "weapons_combined" ); level thread soul_release( self, trig.origin ); return; } Line: if ( ray_gun_hit && doll_hit && flag( "thundergun_hit" ) ) { This states that the ray gun hits once, the doll hits at least once and the thunder gun hits once to activate the end of the Easter egg: level thread soul_release( self, trig.origin ); Explanation: Based on how the code is constructed, this looks more like a bug that wasn't caught in testing. When reviewing it the first time, even I missed the fact that the cross bow wasn't being checked in the last if statement. This was a good find. Thank you for creating the post.
-
What function are you looking in?
-
I'm sorry, but I don't see how this thread has died out. Useful information has been posted here and this thread has been reference in several other topics. As I've said in the past, I'm a Software Engineer with over 5 years of experience. If you or anyone has a valid question about the code or will like to discuss a given topic, then simply ask. -------- New Related Topics: WhosOnFirst post on code relating to the rocket trap that was never fully implemented: http://www.callofdutyzombies.com/forum/viewtopic.php?f=60&t=8302
-
The last 7 posts don't really discuss the topic of this thread. The topic of this tread is to get the lander to fly to another location, station 2. Not to get to another location in the game by glitching. Glitching around doesn't prove anything. The game has to be modded in order to get the lander to fly to station 2. Not by adding a flight path, but by triggering a flight path that already exists. Even if its the starting location that we are flying from; the lander would then fly back there.
-
Alright. I have no idea why others around here at my school say that they where treated poorly but that's what rumors are. I just wanted some facts and you made a good point at the source of that info.
-
I've heard rumors floating around that the programmers where treated rather poorly and that there was a large turn around on programmers. (Programmers are higher-ed and then leave after a short time). Does anyone know anything more about this as I don't have any evidence to support the rumors. One site says that many of the workers treated black ops as a job. http://www.careerbliss.com/company-reviews/black-ops-entertainment-reviews-2317874/review-354547/
-
A thread already exists where you can obtain the code. Note, is only the pre patched code. http://www.callofdutyzombies.com/forum/viewtopic.php?f=60&t=7142
-
I agree completely, this thread has nothing to do with the Easter egg and the ultimate goal is just to see where the lander station would have been.
-
Please remember the purpose of this thread. The point of this is to discuss ideas on how we can access the encrypted script code to trigger the lander's station 2 travel path. We already agree that the location where station 2 my be was never finished. There is a strong possibility that the path was put in when the lander stations where first being developed. This means that if we can trigger this path, such as a call to station 2, then the lander may fly outside of the map to the lander location that people have no clipped to. Keep in mind that the station 2 area was never finished, so the only point of doing this is to prove that the lander location that we can't get to was designed in to the map but then later removed to design changes.
-
There is only one A, it would work if you can use the same letters mulitple times... Treyarch need to some how make it so that one player in solo can complete this easter egg on their own... Make it so you can control where the lander goes in solo, so you can spell out phrases during the lander node. Make the monkey round buttons so they only have to be pressed once in solo. One player needed on the pressure pad in solo. Zeus cannon & the porter x2 raygun for the portal energy. Why make it so it can only be done with 4 in solo???? Regards Snake. I don't know how to replace the existing code but this is how i would make 4 player sync switch made to solo. Here is the existing code: (I formatted it) switch_watcher() { level endon( "between_round_over" ); pressed = 0; switches = GetStructArray( "sync_switch_start", "targetname" ); while (1) { level waittill( "sync_button_pressed" ); timeout = GetTime() + 500; while ( GetTime() pressed = 0; for ( i=0; i if ( IsDefined( switches[i].pressed ) && switches[i].pressed ) { pressed++; } } if ( pressed == 4 ) { flag_set( "switches_synced" ); for ( i=0; i playsoundatposition( "zmb_misc_activate", switches[i].origin ); } return; } wait( 0.05 ); } switch( pressed ) { case 1: case 2: case 3: for ( i=0; i playsoundatposition( "zmb_deny", switches[i].origin ); } break; } for ( i=0; i switches[i].pressed = 0; } } } Line 8: timeout = GetTime() + 500; Changing the 500 to a large number that would still provide a challenge would allow one player to run to all switches before time out. Lline 9: while ( GetTime() Changing it to: "while ( 1 ) {" would force no time out. and would allow the user to press the buttons over the course of the game. This is not efficient as the loop would always have to run. To balance performance, we could remove this: for ( i=0; i switches.pressed = 0; } that way the buttons are never cleared and the time out doesn't matter. So any time the user presses a button, it will add to the total pressed. The buttons could be pressed in different monkey rounds and still count.
-
Very true. I'm going from personal experience as a software engineer. I've been on projects where a design change makes my code needless. Sometimes its left in in case we decide to implement the given feature at a later date.
-
From my understanding, the COD games have a map file with encrypted entities such as vehicles. These vehicles have triggered events that make them follow a predetermined path. I believe the lander is set up the same way. The issue is that these entities are encrypted and are not visible as the event scripts are. According to the viewable script for letter pick ups for Ascension, we are using lander 1, 3, 4, and 5. Lander 2 looks to be removed from the script because of some design change. This means that the trigger to call the lander to station 2 wasn't put in as an entity that you can click on. From a software engineering stand point, it is perfectly reasonable that the code to make the lander to follow a path to station 2 still exists in the lander entity. Does anyone have any ideas on how to activate a vehicle trigger to get it to follow its predetermined path? If anyone knows how to do this, then there will be a good chance we can fly on the lander to station 2. After all, why remove the rout to station two if it could be later brought back in design. level.lander_key[ "lander_station1" ][ "lander_station3" ] = "s"; level.lander_key[ "lander_station1" ][ "lander_station4" ] = "r"; level.lander_key[ "lander_station1" ][ "lander_station5" ] = "e"; level.lander_key[ "lander_station3" ][ "lander_station1" ] = "y"; level.lander_key[ "lander_station3" ][ "lander_station4" ] = "a"; level.lander_key[ "lander_station3" ][ "lander_station5" ] = "i"; level.lander_key[ "lander_station4" ][ "lander_station1" ] = "m"; level.lander_key[ "lander_station4" ][ "lander_station3" ] = "h"; level.lander_key[ "lander_station4" ][ "lander_station5" ] = "u"; level.lander_key[ "lander_station5" ][ "lander_station1" ] = "t"; level.lander_key[ "lander_station5" ][ "lander_station3" ] = "n"; level.lander_key[ "lander_station5" ][ "lander_station4" ] = "l";
-
Yes, I will suggest how others will post on this thread as I created it and am managing it. I believe I answered your first question about the code. If you have any more theories please post them.
-
Please post code in code blocks. This is the section that you where questioning: kill_trig_on_death( trig ) { self waittill( "death" ); trig delete(); if( flag( "thundergun_hit" ) && !flag( "weapons_combined" ) ) { level thread play_egg_vox( "vox_ann_egg6p1_success", "vox_gersh_egg6_fail2", 7 ); } else if( !flag( "weapons_combined" ) ){ level thread play_egg_vox( undefined, "vox_gersh_egg6_fail1", 6 ); } flag_clear( "thundergun_hit" ); } This is the clean up of the Easter egg. So if you die after hitting with the Thunder gun but not the other weapons, vox_gersh_egg6_fail2 will be played. If you didn't hit with the Thunder gun and the weapons didn't all hit. So you throw gursh but don't do anything. Then vox_gersh_egg6_fail1 will play.
-
HITSAM and Continuation to the Nodes Confirmed (VIDEO)
baltostar replied to xStopDropNKillx's topic in Ascension
Like many others when I first started looking at the code and created the questions about the code thread, I too jumped on the HITSAM & HYENA idea that it does something. However rather then just trying it out, I looked at the rest of the code to see where it is used. The spot where it is used doesn't do anything as they are empty if statements. -
Took a double take at what you wrote and had to look at the code again to confirm: level.lander_key[ "lander_station1" ][ "lander_station3" ] = "s"; level.lander_key[ "lander_station1" ][ "lander_station4" ] = "r"; level.lander_key[ "lander_station1" ][ "lander_station5" ] = "e"; level.lander_key[ "lander_station3" ][ "lander_station1" ] = "y"; level.lander_key[ "lander_station3" ][ "lander_station4" ] = "a"; level.lander_key[ "lander_station3" ][ "lander_station5" ] = "i"; level.lander_key[ "lander_station4" ][ "lander_station1" ] = "m"; level.lander_key[ "lander_station4" ][ "lander_station3" ] = "h"; level.lander_key[ "lander_station4" ][ "lander_station5" ] = "u"; level.lander_key[ "lander_station5" ][ "lander_station1" ] = "t"; level.lander_key[ "lander_station5" ][ "lander_station3" ] = "n"; level.lander_key[ "lander_station5" ][ "lander_station4" ] = "l"; As a software engineer, I see the uniformity of the wonders of copy and paste. From the looks of this, it was codded with 5 lander stations. My opinion is that the lines referencing station 2 where removed due to design changes. You know what, assuming my assumption is accurate, then it is reason to believe that there is a design artifact to call the lander to station 2. It is quite possible that the second lander location was never finished but the code in the map may exist for it. If some one can execute a call lander on station 2 with a modified system. There would be a strong possibility that it would fly to that pad that isn't used.
ABOUT US
Call of Duty Zombies (CODZ) is a fan-managed gaming community centered around the popular Call of Duty franchise with central focus on the Zombies mode. Created in 2009, CoDZ is the ultimate platform for discussing Zombies theories, strategies, and connecting players.
Activision, Call of Duty, Call of Duty: Black Ops titles, Call of Duty: Infinite Warfare titles, Call of Duty: WWII are trademarks of Activision Publishing, Inc.
We are not affiliated with Activision nor its developers Treyarch, Sledgehammer, or Infinity Ward.
PARTNERS & AFFILIATES
Interested in becoming an affiliate/partner or looking for business opportunities? Shoot us an email at [email protected] to join the CODZ family. While you're here, show our partners some love!
SITE LINKS
Our most popular pages made convenient for easier navigating. More pages can be found in the navigation menu at the top of the site.